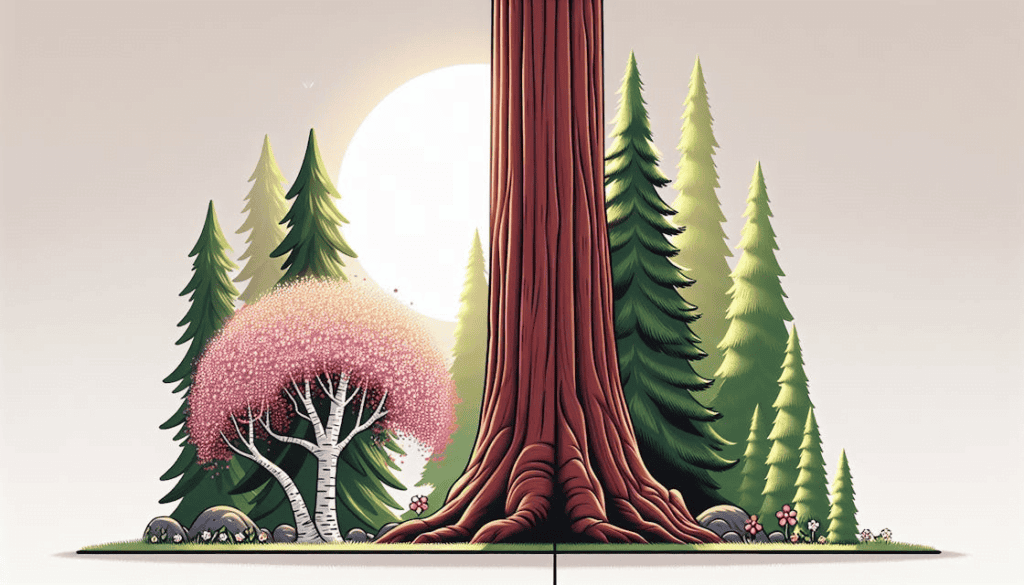
Getting the Hang of Spring Boot
What’s Spring Boot All About?
When I first dipped my toes into Spring Boot, I was blown away by how it makes Java development a breeze. Built on the classic Spring framework, Spring Boot packs all its goodness into a more user-friendly package. Think of it as a microservice wizard that helps you whip up production-ready apps with little to no setup hassle (GeeksforGeeks).
Spring Boot shines when it comes to building REST APIs and can easily turn projects into .war or .jar files for deployment. One of its coolest tricks? Embedded servers like Jetty and Tomcat, so you don’t have to mess around with server setups just to test your app. This makes running and testing your applications a walk in the park.
Feature | What It Does |
---|---|
Base Framework | Built on Spring Framework |
Configuration | Minimal or zero configurations |
Application Type | Stand-alone applications |
Embedded Servers | Jetty, Tomcat, etc. |
File Conversion | .war or .jar files |
Best For | REST APIs, Microservices |
Want more details? Check out the Spring Boot documentation.
Why Spring Boot Rocks
As I dug deeper into Spring Boot, its perks became crystal clear. Here’s why it’s a game-changer:
- Less Boilerplate Code: One of the biggest wins is cutting down on boilerplate code. Spring Boot’s default settings make it super easy to get your app up and running fast (Simplilearn).
- Auto-Configuration Magic: Spring Boot auto-configures your app based on the dependencies you add. This means less time fiddling with settings and more time coding (GeeksforGeeks).
- Embedded Servers: Thanks to embedded servers like Jetty and Tomcat, you can run your apps without setting up a separate server environment (Simplilearn).
- No XML Hassles: Unlike Spring MVC, Spring Boot skips the need for manual XML configuration. This makes setup a breeze (Simplilearn).
- Ready for Production: Spring Boot comes with handy features like metrics, health checks, and externalized configuration, making it easier to keep an eye on your app in a production setting.
For more tips on using Spring Boot for specific tasks, check out our articles on Spring Boot microservices and Spring Boot security.
By tapping into these benefits, Spring Boot has allowed me to build solid, efficient, and production-ready apps with ease. It’s a powerful tool that takes the complexity out of the Spring framework, making it accessible even for newbies like me.
Getting to Know Spring MVC
Spring MVC is a handy tool for developers looking to build solid web applications. Let’s take a closer look at what makes Spring MVC tick and why it’s a go-to choice for many.
What’s Spring MVC All About?
Spring MVC, short for Spring Model-View-Controller, is a Java framework that helps you build web apps by keeping your code organized. It splits your application into three parts: the model, the view, and the controller. This separation makes your code cleaner and easier to manage (Rollbar).
Here’s a quick rundown of the main parts:
- Model: This is where your app’s data lives. It could be a single object or a bunch of them.
- View: This part shows the data to the user. You can use JSP, Thymeleaf, or other template engines to create views.
- Controller: This guy handles user input and updates the model. It’s the middleman between the model and the view.
Spring MVC uses some cool features from the Spring framework, like Inversion of Control (IoC) and Dependency Injection (DI), to make building web apps a breeze (GeeksforGeeks).
What Makes Spring MVC Special?
Spring MVC packs a bunch of features that make web development easier. Here are some highlights:
- Annotation-Based Configuration:
- You can use annotations to set up controllers and request mappings, which makes your code cleaner.
- Examples include
@Controller
,@RequestMapping
, and@GetMapping
.
- Handler Mapping:
- This maps HTTP requests to the right handler methods.
- You can set it up using annotations or XML.
- View Resolvers:
- These decide which view to show based on what the controller returns.
- Common ones are
InternalResourceViewResolver
for JSP andThymeleafViewResolver
for Thymeleaf.
- Form Handling:
- Makes processing forms easier with data binding, validation, and form tags.
- Use
@ModelAttribute
to bind form data to model objects.
- Exception Handling:
- Has a solid way to handle exceptions.
- Use
@ExceptionHandler
to create custom exception handling methods.
- Internationalization (i18n):
- Built-in support for making your app multilingual.
- Easy to create web apps that speak multiple languages.
- Integration with Other Spring Modules:
- Works smoothly with other Spring modules like Spring Security, Spring Data, and Spring Boot.
Here’s a quick table to sum up the key features:
Feature | What It Does |
---|---|
Annotation-Based Config | Makes setting up controllers and request mappings easier |
Handler Mapping | Maps HTTP requests to the right methods |
View Resolvers | Decides which view to show |
Form Handling | Simplifies form processing with data binding and validation |
Exception Handling | Solid way to handle exceptions |
Internationalization | Supports creating multilingual web apps |
Integration | Works well with other Spring modules |
Spring MVC is a great choice for building traditional web apps. Its features and integration options make it a favorite among Java developers. Want to know more about how Spring MVC stacks up against Spring Boot? Check out our article on spring boot vs spring mvc.
For more tips and tricks, you might also like our spring boot tutorial and spring boot documentation.
Comparing Spring Boot and Spring MVC
Getting to Know Spring Boot
Spring Boot is like the cool, laid-back cousin of the traditional Spring framework. It makes life easier by cutting down on the setup and letting you dive straight into building your app. Perfect for whipping up REST APIs in no time.
One of the best things about Spring Boot is its auto-configuration magic. No more fiddling with endless settings—just focus on your code. Plus, you can package your projects into WAR or JAR files, making deployment a breeze.
Spring Boot also comes with built-in servers like Jetty and Tomcat, so you don’t have to mess around setting up a server for testing. It’s a dream for anyone who wants to get started quickly. For a step-by-step guide, check out our spring boot tutorial.
Here’s a snapshot of what Spring Boot brings to the table:
Feature | What It Does |
---|---|
Auto-Configuration | Sets up your app based on the dependencies you add. |
Embedded Servers | Includes servers like Jetty and Tomcat for easy testing and deployment. |
Minimal Configuration | Cuts down on boilerplate code and skips the XML hassle. |
Production-Ready | Offers metrics, health checks, and more right out of the box. |
Spring MVC vs. Spring Boot
Both Spring MVC and Spring Boot are part of the Spring family, but they serve different needs.
Spring MVC is your go-to for building web applications. It requires you to roll up your sleeves and set up everything manually, including XML files. This can be a bit of a slog, but it gives you fine-grained control over your app.
Spring Boot, on the other hand, is all about making your life easier. It’s a module of the Spring framework that lets you build stand-alone apps with little to no configuration. It trims down the boilerplate code and offers default settings to get you up and running fast. This makes testing a walk in the park.
Here’s a side-by-side comparison:
Aspect | Spring MVC | Spring Boot |
---|---|---|
Configuration | Manual setup, including XML | Auto-configured, minimal setup |
Embedded Servers | Needs explicit server setup | Comes with embedded servers like Jetty, Tomcat |
Use Case | Best for traditional web apps | Ideal for microservices and REST APIs |
Deployment | Uses WAR files | Can use WAR or JAR files |
Boilerplate Code | More boilerplate | Less boilerplate |
Testing | More complex setup | Easier and faster |
If you’re just starting out, Spring Boot is usually the way to go because it’s simpler and quicker to use. It’s especially great for building microservices, as we explain in our spring boot microservices guide. But if you’re working on a more complex web app that needs detailed control, Spring MVC might be your best bet.
In a nutshell, knowing the differences between Spring Boot and Spring MVC helps you choose the right tool for your project. Whether you want ease of development or more control, both frameworks have their perks. For more info, check out the spring boot documentation and explore topics like spring boot security.
Practical Applications and Considerations
When to Use Spring Boot
If you’re a junior Java developer, you might be scratching your head about when to use Spring Boot. Here are some common scenarios where Spring Boot really shines:
- Quick App Development: Spring Boot’s straightforward approach makes it perfect for whipping up applications fast. Its auto-configuration feature cuts down on the manual setup, letting you dive right into writing business logic. For a step-by-step guide, check out our Spring Boot tutorial.
- Microservices: Spring Boot is a top pick for microservices. Its lightweight nature and support for embedded servers make deploying microservices a breeze. You can dig deeper into this in our article on Spring Boot microservices.
- Cloud Deployments: If you’re working on cloud-based apps, Spring Boot integrates smoothly with cloud platforms. Its ease of use and production-ready features make it a go-to for cloud-native development.
- Backend Development: Spring Boot is built for backend tasks, offering a solid framework for web development. Its auto-configuration handles most of the setup, so you can jump straight into coding (Quora).
Choosing Between Spring Boot and Spring MVC
Deciding between Spring Boot and Spring MVC can be tricky. Here are some tips to help you choose:
- Ease of Setup: Spring Boot needs fewer configurations compared to Spring MVC. This makes it quicker to get started, especially if you’re new to Spring. Its auto-configured nature means you don’t need to manually set up many components (Quora).
- Speed of Development: If you need to quickly develop and deploy an app, Spring Boot is the way to go. Its opinionated defaults and pre-configured setups cut down development time significantly. For more details, check out the Spring Boot documentation.
- Production-Ready Features: Spring Boot comes with built-in production-ready features like health checks, metrics, and externalized configuration. These features are essential for deploying apps in a production environment without needing a ton of extra setup.
- Flexibility and Customization: While Spring Boot is super convenient for most projects, Spring MVC offers more flexibility and customization. If you have specific needs that demand fine-grained control over your configuration, Spring MVC might be the better choice.
Here’s a quick comparison to sum it up:
Feature | Spring Boot | Spring MVC |
---|---|---|
Configuration | Minimal | Extensive |
Development Speed | Fast | Moderate |
Production-Ready | Yes | No (needs extra setup) |
Flexibility | Moderate | High |
For more on securing your Spring Boot app, visit our guide on Spring Boot security. If you need more tips on choosing the right framework, don’t miss our article on tips for choosing between Spring Boot and Spring MVC.