Introduction
Programming languages form the backbone of modern technology, acting as the formal systems through which humans communicate instructions to computers. Whether it’s developing software, controlling hardware, or building entire digital ecosystems, programming languages are essential for translating complex human logic into actions that machines can execute.
This blog will explore what defines a programming language, highlighting its key components and features. By examining the fundamental elements of programming languages and categorizing them based on their levels of abstraction and usage, we aim to provide a comprehensive understanding for both beginners and seasoned developers. Through this detailed exploration, readers will gain insights into why programming languages are structured as they are and how to choose the right one for their needs.
Understanding programming languages is crucial for anyone interested in technology, computer science, or software development. With the rapid evolution of the digital landscape, grasping the nuances of these languages not only empowers individuals to create innovative solutions but also demystifies the seemingly complex world of coding. By the end of this blog, you’ll have a clearer picture of what constitutes a programming language and why they are indispensable tools in the tech world.
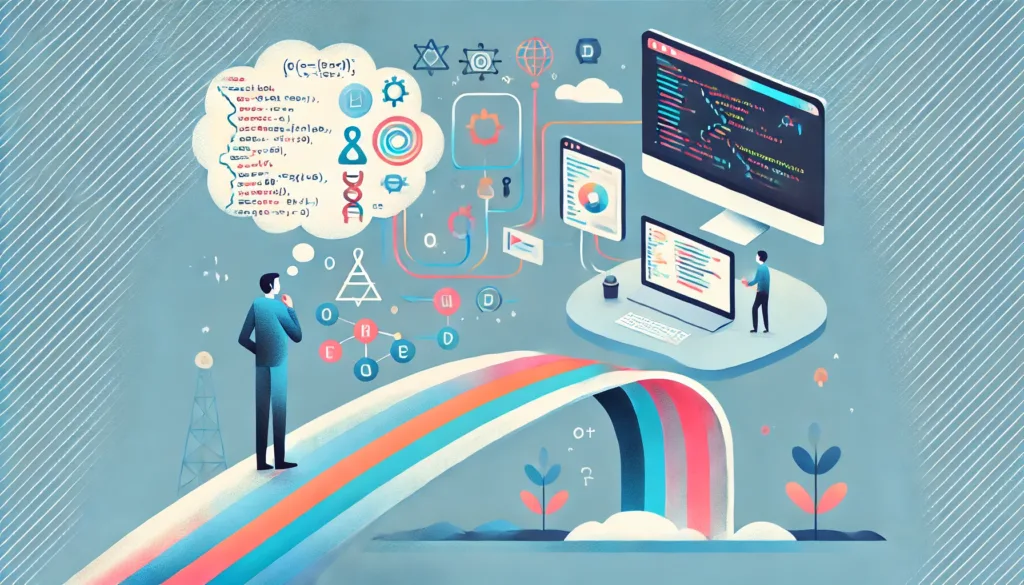
Definition and Purpose of Programming Languages
What is a Programming Language?
A programming language is a formal set of rules and syntax used to communicate instructions to a computer. It serves as a critical bridge between human logic and machine operations, enabling developers to write code that can perform a wide range of tasks — from simple computations to complex algorithms. By defining both the structure and meaning of statements, programming languages allow for precise and unambiguous communication between the programmer and the machine.
In essence, a programming language translates human ideas into a format that computers can process, making it possible to create everything from mobile applications and games to complex artificial intelligence systems. Without programming languages, harnessing the full power of computers would be nearly impossible, as machines can only interpret very specific, logical instructions.
Why Do We Need Programming Languages?
Programming languages are essential because they provide a structured way for humans to communicate with machines. Unlike humans, computers don’t understand natural language — they require commands that are meticulously organized and adhere to strict rules. While natural language is flexible and often context-dependent, computers require precision and clarity to execute instructions correctly.
Programming languages fill this gap by offering a controlled medium through which complex tasks can be broken down into a series of smaller, well-defined steps. This need for structure is why programming languages exist: they translate high-level human concepts into low-level machine actions. They allow developers to express ideas in a way that is not only logical and coherent for computers but also readable and maintainable for other humans.
Key Features of a Programming Language
1. Syntax
Syntax refers to the set of rules that dictate the structure of statements within a programming language. Just as grammar governs sentence construction in natural languages, syntax defines how symbols, keywords, and operators must be arranged for the code to be considered valid. Syntax ensures that instructions are written in a way that the compiler or interpreter can parse and execute without errors.
For instance, in Python, a simple conditional statement might look like this:
if x > 10:
print("x is greater than 10")
In this example, the if
keyword initiates a conditional statement, and the colon (:
) signals the start of the code block to be executed if the condition is true. If any of these elements are misplaced or omitted, the syntax rules of the language will flag an error.
2. Semantics
While syntax focuses on the form of a statement, semantics is concerned with its meaning. In programming, semantics determines what actions a syntactically correct statement performs. Two pieces of code may be syntactically correct but semantically different, depending on how the program interprets them.
For example, consider the following two Python statements:
print("5" + "5") # Outputs: 55
print(5 + 5) # Outputs: 10
Although both statements use the +
operator, their meanings differ. The first statement concatenates two strings (“5” and “5”), resulting in “55”, while the second statement adds two integers (5 and 5), producing 10. Thus, semantics governs how data types, operators, and functions interact to produce a specific outcome.
3. Data Types
Data types are classifications that specify the kind of data that can be stored and manipulated within a program. They dictate the operations that can be performed on the data and the way it is stored in memory. The most common data types include:
- Integers: Whole numbers, such as
42
or-7
. - Strings: Sequences of characters, like
"Hello, World!"
. - Booleans: Logical values,
True
orFalse
.
For example, in Python, data types can be defined and used as follows:
age = 30 # Integer
name = "Alice" # String
is_student = True # Boolean
Each of these variables is assigned a different data type, and operations performed on them depend on their type. For instance, trying to add an integer to a string will result in a type error, demonstrating how data types govern the interactions between different elements in code.
4. Control Structures
Control structures are constructs that direct the flow of execution in a program, allowing developers to implement complex logic through decisions and iterations. Common control structures include:
- Conditional Statements:
if
,else
, andelif
clauses that decide which code block to execute based on a condition. - Loops:
for
andwhile
loops that repeat a code block until a certain condition is met.
For example, a simple for
loop in Python that prints numbers from 1 to 5 looks like this:
for i in range(1, 6):
print(i)
The loop iterates over the range from 1 to 5, printing each number in sequence. Control structures are fundamental in defining the logic of programs, determining which parts of the code should run under various conditions, and ensuring that the program behaves predictably.
5. Abstraction
Abstraction is a core principle in programming that helps manage complexity by hiding low-level details and exposing only the necessary functionality. It allows developers to focus on high-level logic without worrying about the inner workings of specific code segments. This approach makes programs more modular and easier to maintain.
For example, in object-oriented programming (OOP), classes provide a form of abstraction:
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
def start(self):
print(f"{self.make} {self.model} is starting...")
# Using the abstraction without needing to know internal details
my_car = Car("Toyota", "Corolla")
my_car.start()
In this case, the internal implementation of how the Car
class starts is abstracted away, and the user only interacts with the start()
method. Abstraction is crucial for building large-scale systems, as it allows developers to build complex applications with simpler, well-defined interfaces.
6. Libraries and Frameworks
Libraries and frameworks are collections of pre-written code that provide standardized ways to accomplish common tasks, such as interacting with databases, building user interfaces, or performing mathematical computations. They simplify the development process by offering reusable components, enabling programmers to focus on the unique aspects of their application rather than reinventing the wheel.
For instance, Python’s pandas
library is widely used for data manipulation, while the Flask
framework helps developers create web applications. Similarly, JavaScript’s React
framework is popular for building dynamic user interfaces, and Java’s Spring
framework is extensively used for enterprise-level applications.
Here’s a small example of using a library in Python:
import pandas as pd
# Using the pandas library to create a simple DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
df = pd.DataFrame(data)
print(df)
In this example, the pandas
library simplifies the creation and manipulation of a data table, demonstrating the power of libraries in streamlining coding tasks. Frameworks, on the other hand, often provide a more extensive structure and set of rules for building applications, making them ideal for complex projects.
Different Types of Programming Languages
1. Low-Level Languages
Low-level programming languages are closer to the hardware and machine architecture, providing minimal abstraction from the computer’s physical components. These languages are directly translated into machine instructions, making them highly efficient but also challenging to work with. They require a deep understanding of computer hardware, memory management, and instruction sets.
Examples:
- Machine Language: This is the lowest level of programming, consisting entirely of binary code (0s and 1s). Each instruction corresponds directly to a specific operation in the CPU. Machine language is rarely written by humans, as it is highly error-prone and difficult to debug.
- Assembly Language: Assembly is a step above machine language, using mnemonic codes (such as
MOV
,ADD
, andSUB
) instead of binary. While still hardware-specific, assembly language is slightly more readable and often used for system programming, hardware control, and performance-critical tasks. Developers use assembly when they need fine control over the hardware, such as in embedded systems or game engines.
2. High-Level Languages
High-level programming languages are designed to be more user-friendly, with greater abstraction from the underlying hardware. They use natural language elements and logical structures, making them easier to read, write, and maintain. High-level languages handle many low-level details automatically, such as memory management, enabling developers to focus more on logic and functionality.
You can learn more about high level languages in this post if you are curious about them!
Advantages:
- Improved readability and simplicity.
- Easier to learn and use, even for beginners.
- Faster development time due to automated features like garbage collection.
- Platform independence — most high-level languages can be compiled or interpreted to run on various systems.
Examples:
- Python: Known for its simplicity and readability, Python is often used in data science, machine learning, and web development.
- Java: A versatile language with a “write once, run anywhere” philosophy, Java is used in enterprise applications, Android development, and large-scale systems.
- C++: A powerful language that offers both low-level control and high-level abstractions, C++ is popular in game development, system software, and performance-critical applications. If you think C++ is hard, you need to read this article now.
3. Scripting Languages
Scripting languages are typically high-level languages that are interpreted rather than compiled. They are often used for automating repetitive tasks, manipulating text, and enhancing the functionality of existing systems. Scripting languages are designed for quick and easy development, making them ideal for tasks that don’t require the complexity or performance of compiled languages.
Typical Use Cases:
- Web Development: Scripting languages like JavaScript are essential for adding interactivity and dynamic content to web pages.
- Task Automation: Languages such as Python are frequently used to automate tasks like file manipulation, web scraping, or data processing.
Examples:
- JavaScript: Primarily used for client-side web development, JavaScript enables dynamic content and interactive features on websites.
- Python: While often used as a general-purpose programming language, Python also excels in scripting tasks, such as automating workflows and testing.
4. Domain-Specific Languages (DSLs)
Domain-Specific Languages (DSLs) are specialized programming languages designed to solve problems within a specific domain or industry. Unlike general-purpose languages, DSLs provide constructs and syntax tailored to a particular set of tasks, making them highly efficient for domain-specific problems but less versatile outside their niche.
The goal of a DSL is to simplify complex operations within a given domain, allowing developers and non-developers alike to express concepts more concisely. By providing a focused syntax and set of rules, DSLs reduce complexity and make it easier to implement domain-specific solutions.
Examples:
- SQL (Structured Query Language): A DSL designed for managing and querying relational databases. SQL allows users to retrieve and manipulate large datasets with simple commands like
SELECT
,INSERT
, andUPDATE
. - HTML (HyperText Markup Language): Used for structuring and displaying content on the web, HTML defines the elements (e.g., paragraphs, headings, images) that compose a webpage.
- MATLAB: A DSL for mathematical computations, simulations, and data visualization, widely used in engineering and scientific research.
Looking for the best programming languages to learn? You can check this article to learn more!
Criteria for Considering a System a Programming Language
Not every system that facilitates communication with a computer can be classified as a programming language. To be considered a true programming language, a system must possess a few fundamental characteristics. These core elements ensure that the language can effectively communicate complex instructions to a computer, support logical constructs, and execute tasks reliably. The criteria for defining a programming language include:
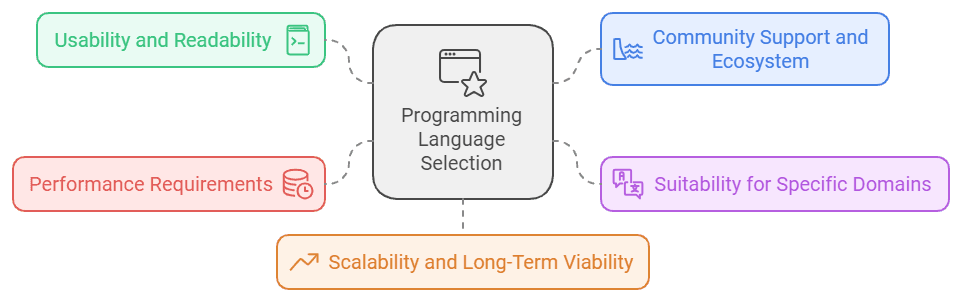
1. Formal Syntax
Syntax refers to the formal set of rules that govern how statements and expressions must be structured in a programming language. A valid programming language must have a clearly defined syntax that dictates how symbols, keywords, and operators should be combined to form instructions that the system can parse. Without formal syntax, it would be impossible to distinguish between valid and invalid commands, leading to confusion for both the compiler/interpreter and the programmer.
For example, in Python, the syntax rule for defining a function is:
def function_name(parameters):
# Function body
The absence of any element (such as the def
keyword or the colon :
) would make the code invalid. Thus, syntax rules are the foundation that ensures code is properly formatted and interpretable.
2. Defined Semantics
Semantics refer to the meaning behind syntactically correct statements. While syntax tells a computer how to interpret a statement’s structure, semantics define what the statement should do when executed. A programming language must have well-defined semantics to ensure that the intended actions are carried out correctly.
For example, consider two similar statements:
print(5 + 5) # Outputs: 10
print("5" + "5") # Outputs: 55
Both lines are syntactically correct, but their semantics differ based on the data types involved. In the first statement, the +
operator is used to perform arithmetic addition, while in the second statement, it is used for string concatenation. Clear semantics help prevent ambiguity and ensure that developers know exactly how their code will behave.
3. Ability to Perform Computations
To be considered a programming language, a system must support the ability to perform basic and complex computations. This includes operations such as arithmetic calculations, data processing, and logical operations. Without this capability, the language would be limited to simple declarative statements without the power to perform meaningful tasks.
For instance, even the simplest programming languages must support basic arithmetic operations like addition, subtraction, multiplication, and division. More advanced languages provide extensive computational features, such as matrix manipulations, data transformations, and algorithmic problem-solving, making them suitable for various applications.
4. Control Structures
Control structures are essential components that guide the flow of a program. These structures include conditional statements (e.g., if-else
), loops (e.g., for
and while
), and function calls. They enable the programmer to define the sequence in which code is executed, implement decision-making logic, and repeat instructions based on specific conditions.
For example, a simple loop in Python looks like this:
for i in range(5):
print(i)
This for
loop iterates five times, printing the values 0 through 4. Without control structures, programming languages would be limited to executing instructions in a linear and sequential manner, greatly restricting their usefulness for real-world problem solving.
If you’d like to learn loops in Java, you can read this article!
By meeting these core criteria—formal syntax, defined semantics, ability to perform computations, and control structures—a system can be classified as a programming language, capable of transforming high-level ideas into executable code.
Choosing the Right Programming Language
Selecting the right programming language is a critical decision that can impact the efficiency, scalability, and maintainability of a project. Each language comes with its own strengths, weaknesses, and ideal use cases. Understanding the various factors that influence this choice can help developers and businesses pick the language best suited to their needs.
1. Factors to Consider
Usability and Readability
Usability refers to how easy it is for developers to write, read, and maintain code in a given language. Languages with simple syntax, such as Python, are often preferred for their readability, making them ideal for beginners and for collaborative projects where multiple developers are involved. Additionally, languages that emphasize readability reduce the likelihood of bugs and make the codebase easier to navigate.
Community Support and Ecosystem
The size and engagement of a language’s community play a crucial role in its viability. A robust community means access to a wealth of libraries, tools, and frameworks, as well as active forums and resources for troubleshooting. For example, JavaScript has a vast community with numerous frameworks like React and Node.js, making it an excellent choice for web development.
Suitability for Specific Domains
The nature of the project will heavily influence the language choice. Some languages are tailored for specific domains, offering features and tools that simplify development in those areas:
- Data Science and Machine Learning: Python is the dominant language due to its extensive libraries (e.g., Pandas, NumPy, Scikit-learn) and user-friendly syntax.
- Web Development: JavaScript is essential for front-end development, while languages like Ruby and PHP are popular for backend logic.
- System Programming: C and C++ provide low-level memory access, making them ideal for operating system development and performance-critical applications.
- Mobile Development: Swift (iOS) and Kotlin (Android) are optimized for building native mobile applications.
Performance Requirements
Some projects require high computational efficiency and low-level memory management, in which case performance-oriented languages like C++ or Rust may be preferred. Conversely, for projects where development speed is more critical than raw performance, high-level languages like Python or JavaScript are more suitable.
Scalability and Long-Term Viability
Choosing a language that can scale with the project’s needs and is likely to remain relevant is also crucial. Java, for instance, is a language with a strong track record of backward compatibility and long-term support, making it a reliable choice for large-scale enterprise systems.
2. Practical Examples
- Building a Web Application
For full-stack web applications, developers often choose a combination of JavaScript for front-end interactivity (using frameworks like React or Vue.js) and a backend language like Python (with Flask or Django) or JavaScript (using Node.js). This combination allows for seamless integration between the client and server-side code. - Developing a Machine Learning Model
Python is the go-to choice due to its rich ecosystem of data science libraries. For instance, a machine learning project might involve using Scikit-learn for model building, Pandas for data manipulation, and Matplotlib for data visualization. - Creating a Mobile App
For native mobile app development, Swift is the preferred language for iOS apps due to its tight integration with Apple’s ecosystem, while Kotlin is favored for Android development because of its modern features and Google support. For cross-platform development, languages like Dart (with Flutter) or JavaScript (with React Native) are popular. - Building a High-Performance Game Engine
C++ is often used for game engine development because it offers fine-grained control over hardware resources and memory, making it ideal for performance-critical applications like 3D graphics rendering.
By aligning language choices with project goals, developers can leverage the strengths of each language to optimize productivity and performance.
Conclusion
Programming languages are the tools that turn human logic into machine action, serving as the foundation for every software application, algorithm, and digital innovation. This blog explored what defines a programming language, the essential features they must possess, and the different types available. We also discussed the factors to consider when choosing the right language and provided practical examples to guide decision-making.
Selecting the appropriate programming language can streamline development, enhance project outcomes, and ensure long-term viability. The diversity of languages—ranging from low-level systems programming to high-level web scripting—enables developers to tailor their tools to their specific needs. By understanding the unique strengths and ideal use cases of each language, developers can build more effective and elegant solutions.
Ultimately, the choice of a programming language should reflect the goals of the project, the expertise of the development team, and the needs of the target audience. Exploring different languages not only broadens one’s technical repertoire but also deepens the understanding of how software development shapes the digital world.
FAQ: Frequently Asked Questions
1. What is the difference between a programming language and a scripting language?
A programming language is a broader category that includes both compiled and interpreted languages used to build software applications and systems. Scripting languages, on the other hand, are typically used for automating tasks, enhancing existing applications, and are often interpreted rather than compiled. Examples of scripting languages include Python and JavaScript.
2. How do I decide which programming language to learn first?
Your choice should depend on your goals and interests. For general-purpose programming and ease of learning, Python is a great starting point due to its simplicity and versatility. If you are interested in web development, JavaScript is essential. For those looking into game development or systems programming, C++ or C# would be a good fit.
3. What are low-level languages used for?
Low-level languages, such as machine language and assembly, are used when direct hardware manipulation and high performance are required. They are commonly found in systems programming, embedded systems, and scenarios where efficiency is crucial, like in the development of operating systems or game engines.
4. Can a programming language belong to more than one category?
Yes, some languages can fit into multiple categories depending on how they are used. For example, Python is considered both a high-level language and a scripting language because it can be used for building complete applications as well as for scripting and automation.
5. What is the main factor that differentiates one programming language from another?
The primary differentiators are syntax, semantics, and purpose. Some languages are designed for ease of use and readability (like Python), while others focus on performance and control (like C++). Additionally, the presence of specialized libraries and frameworks tailored to specific tasks also sets them apart.
References
- Wikipedia: Programming Languages
- GeeksforGeeks: Introduction to Programming Languages
- Britannica: Computer Programming Language
- Code Institute: What is a Programming Language?
- Study.com: Programming Language Types & Examples
- JavaTpoint: Programming Language
- Codecademy: What is a Programming Language?
- Unstop: What is a Programming Language?
These references provide a comprehensive overview of programming languages, their definitions, key features, and types, supporting the content covered in the blog.