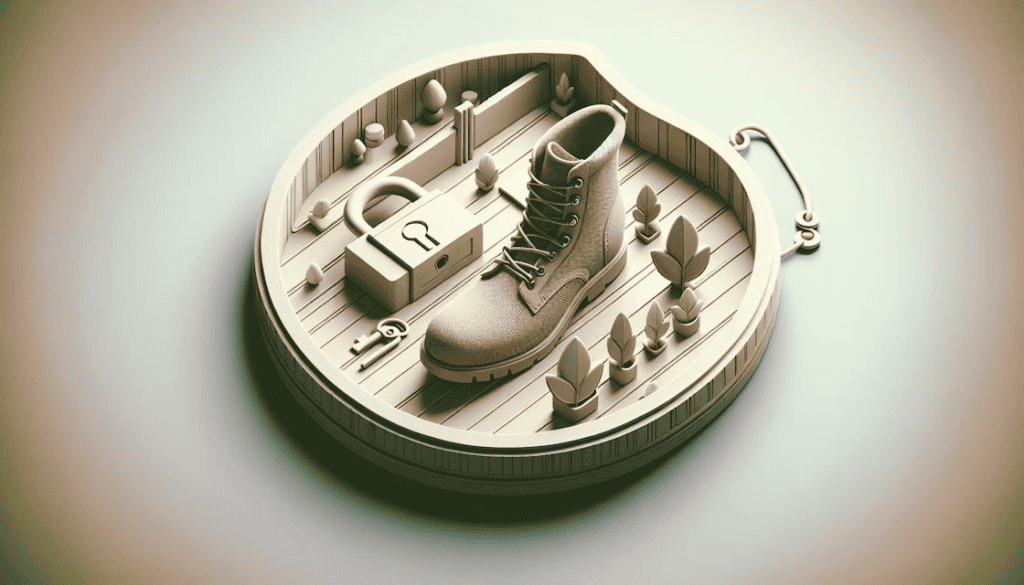
Getting the Hang of Spring Boot Security
When you’re diving into Spring Boot, getting a grip on security is a must. Let’s break it down and get you up to speed.
What’s Spring Security All About?
Spring Security is like your app’s bouncer. It handles who gets in and what they can do once inside. It’s built to fend off common attacks like Cross-Site Request Forgery (CSRF). This framework takes care of both who can access your web pages and who can call your methods (GeeksforGeeks).
Spring Security is packed with features to cover all your security needs:
- Authentication: Who are you?
- Authorization: What can you do?
- Exploit Protection: Keeping the bad guys out
It also plays nice with other libraries, making it easier to use (Spring Security Reference).
If you’re just starting out with Spring Boot, check out this spring boot tutorial for a solid intro.
Key Ideas Behind Spring Security
Spring Security is built on a few key ideas that make it a strong choice for securing your apps:
- Authentication: Proving who you are. This can be done through form logins, OAuth 2.0, and more.
- Authorization: Deciding what you can do. This includes role-based access and method-level security.
- Exploit Protection: Guarding against common attacks like CSRF.
- Integration: Working smoothly with other Spring projects and libraries.
Spring Security offers cool features like single sign-on, “remember-me” options, and various ways to log in, like LDAP and JAAS (GeeksforGeeks).
Concept | What It Does | Example Annotations |
---|---|---|
Authentication | Proving who you are | @PreAuthorize |
Authorization | Deciding what you can do | @Secured |
Method Security | Controlling access to methods based on roles or permissions | @RolesAllowed |
Exploit Protection | Guarding against vulnerabilities like CSRF | @PostAuthorize |
Spring Security also has advanced features like OAuth 2.0 login, letting users log in with GitHub or Google accounts, and reactive support for Spring WebFlux (GeeksforGeeks).
For more on Spring Boot, check out our articles on spring boot microservices and spring boot vs spring mvc. For detailed documentation, head over to the spring boot documentation.
Making Spring Security Work in Spring Boot
Getting Spring Security up and running in your Spring Boot app isn’t rocket science once you get the hang of it. Let’s break it down into bite-sized pieces: authentication, authorization, and the user entity with the UserDetails interface.
Authentication and Authorization
These two are the gatekeepers of your app. Authentication checks who you are, while authorization decides what you’re allowed to do.
Authentication: Spring Security uses a filter to catch requests and check user credentials. If you’re good to go, it sends you to the page you wanted in the first place. To set this up, you need a UserDetailsService and a PasswordEncoder.
Authorization: This is where you set the rules. With Spring Security, you can use annotations like @PreAuthorize
, @PostAuthorize
, @Secured
, and @RolesAllowed
to control who gets to do what.
Here’s a quick example of setting up role-based access:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasRole("USER")
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
For more details, check out our Spring Boot security guide.
User Entity and UserDetails Interface
The user entity is the heart of your authentication system. It represents the user and implements the UserDetails interface from Spring Security. This interface includes properties like id
, username
, password
, and role
.
Here’s a simple user entity:
@Entity
public class User implements UserDetails {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String password;
private String role;
@Override
public Collection<? extends GrantedAuthority> getAuthorities() {
return Collections.singletonList(new SimpleGrantedAuthority(role));
}
@Override
public String getPassword() {
return password;
}
@Override
public String getUsername() {
return username;
}
@Override
public boolean isAccountNonExpired() {
return true;
}
@Override
public boolean isAccountNonLocked() {
return true;
}
@Override
public boolean isCredentialsNonExpired() {
return true;
}
@Override
public boolean isEnabled() {
return true;
}
}
To load user data, you need to implement the UserDetailsService interface. This service fetches user details by username and uses a PasswordEncoder to hash passwords.
Here’s an example:
@Service
public class MyUserDetailsService implements UserDetailsService {
@Autowired
private UserRepository userRepository;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userRepository.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found");
}
return user;
}
}
To keep your Spring Boot app secure, follow these steps carefully. For more tips and tricks, check out our Spring Boot tutorial.
Advanced Features of Spring Security
Spring Security packs a punch with features that can seriously beef up your Spring Boot apps. Let’s talk about OAuth 2.0 Login, Reactive Support, and Modernized Password Encoding.
OAuth 2.0 Login
OAuth 2.0 Login is a game-changer in Spring Security 6.0. It lets users log in using their existing accounts from big names like GitHub or Google. This not only makes the login process smoother but also taps into the robust security of these providers.
To get OAuth 2.0 Login rolling in your Spring Boot app, add this to your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-oauth2-client</artifactId>
</dependency>
Then, configure it in your application.yml
:
spring:
security:
oauth2:
client:
registration:
google:
client-id: YOUR_CLIENT_ID
client-secret: YOUR_CLIENT_SECRET
scope: profile, email
redirect-uri: "{baseUrl}/login/oauth2/code/{registrationId}"
provider:
google:
authorization-uri: https://accounts.google.com/o/oauth2/auth
token-uri: https://oauth2.googleapis.com/token
user-info-uri: https://www.googleapis.com/oauth2/v3/userinfo
For a step-by-step guide, check out the Spring Boot Documentation.
Reactive Support and Password Encoding
Reactive Support
Spring Security 6.0 also brings reactive programming into the mix, making it a perfect fit with Spring WebFlux. If your app needs to handle a ton of requests without breaking a sweat, this is for you.
Here’s a basic setup for reactive security:
@EnableWebFluxSecurity
public class SecurityConfig {
@Bean
public SecurityWebFilterChain securityWebFilterChain(ServerHttpSecurity http) {
return http
.authorizeExchange()
.pathMatchers("/login", "/public/**").permitAll()
.anyExchange().authenticated()
.and()
.oauth2Login()
.and()
.build();
}
}
For more details, visit our Spring Boot Microservices guide.
Password Encoding
Spring Security’s modernized password encoding uses the DelegatingPasswordEncoder
, which supports multiple encoding methods. This means even if you change how passwords are encoded, old ones will still work.
To set up password encoding:
@Bean
public PasswordEncoder passwordEncoder() {
return PasswordEncoderFactories.createDelegatingPasswordEncoder();
}
Or, if you want to specify different encoders:
@Bean
public PasswordEncoder passwordEncoder() {
Map<String, PasswordEncoder> encoders = new HashMap<>();
encoders.put("bcrypt", new BCryptPasswordEncoder());
encoders.put("scrypt", new SCryptPasswordEncoder());
return new DelegatingPasswordEncoder("bcrypt", encoders);
}
For more on password encoding and security best practices, check out Spring Boot vs Spring MVC.
By using these advanced features, you can make your Spring Boot apps more secure and resilient against various threats.
Best Practices for Spring Boot Security
Keeping your Spring Boot app secure is a must. By sticking to some tried-and-true practices, you can protect your app from nasty surprises. Let’s talk about two biggies: HTTPS and CSRF protection.
HTTPS Implementation
HTTPS is like a security blanket for your app. It scrambles data during transmission, so snoopers can’t read sensitive info. This is a no-brainer for apps dealing with personal data.
To get HTTPS up and running in your Spring Boot app, you need an SSL certificate. Here’s a quick setup:
server:
ssl:
key-store: classpath:keystore.p12
key-store-password: changeit
key-store-type: PKCS12
key-alias: tomcat
port: 8443
Configuration | Description |
---|---|
key-store | Path to the keystore file |
key-store-password | Password for the keystore |
key-store-type | Type of keystore (e.g., PKCS12) |
key-alias | Alias for the key |
port | Port number for HTTPS |
Don’t forget to regularly test your app for security holes. Use Spring Boot’s testing tools or a dedicated API tester like Escape (Escape Tech). For more details, check out the Spring Boot documentation.
Cross-Site Request Forgery (CSRF) Protection
CSRF attacks are sneaky. They trick users into doing stuff they didn’t mean to, which can mess up your app’s security. Spring Security has CSRF protection on by default, but you should double-check it’s set up right.
CSRF protection works by adding a unique token for each session. This token must be included in any request that changes data on the server. Here’s how to make sure CSRF protection is on:
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf()
.csrfTokenRepository(CookieCsrfTokenRepository.withHttpOnlyFalse());
}
Using CookieCsrfTokenRepository
stores the CSRF token in a cookie, making it easy to include in requests. This setup helps fend off CSRF attacks without making things harder for users.
Feature | Description |
---|---|
csrfTokenRepository | Repository for storing CSRF tokens |
withHttpOnlyFalse | Makes the CSRF token accessible to JavaScript |
Regularly review and test your CSRF setup to make sure it’s working right (Snyk). For more tips on boosting your app’s security, check out our Spring Boot tutorial.
By following these tips, you can make your Spring Boot app a lot more secure. Stay on top of the latest security trends and keep an eye on your app to keep it safe.