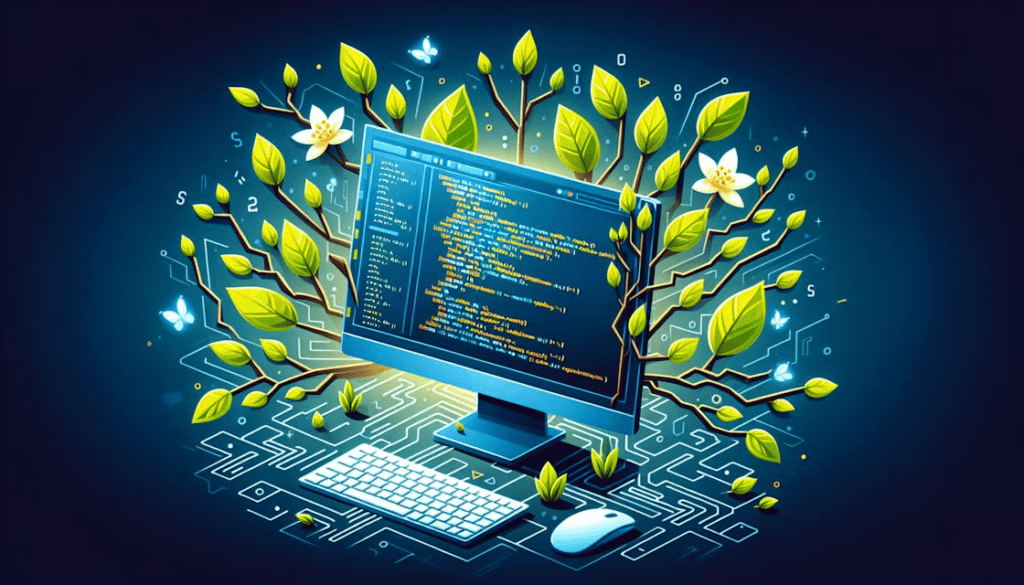
Introduction to Spring Boot
Getting the Hang of Spring Boot
When I first dipped my toes into Java development, Spring Boot quickly became my trusty sidekick. It’s designed to make building web apps and microservices with the Spring Framework a breeze (IBM). Here’s why it rocks:
- Autoconfiguration: Spring Boot sets up your app based on the stuff you’ve added. No need to fiddle with endless settings.
- Opinionated Defaults: It gives you a head start with default settings, so you don’t get lost in a sea of options.
- Stand-Alone Apps: Your app can run on its own without needing an external server. Just click and go.
Spring Boot sits on top of the regular Spring Framework, packing all its goodies but making it way easier to use. It’s a lifesaver for whipping up production-ready apps, especially when you’re dealing with REST APIs (GeeksforGeeks).
Why Spring Boot is Awesome
As a newbie Java developer, Spring Boot has been a game-changer for me:
- Simplicity: The biggest win is how simple it makes things. With auto-configuration, I can focus on writing the actual code instead of getting bogged down with setup. This is a godsend for complex projects.
- Microservices Made Easy: Spring Boot’s self-contained apps are perfect for microservices. Each service runs on its own, making deployment and scaling a cinch (GeeksforGeeks). Check out my spring boot microservices guide for more.
- Built-In Server: No need to mess around with setting up an external server. Spring Boot apps come with an embedded server, making deployment a no-brainer.
- No Boilerplate Code: Forget about generating code or dealing with XML configurations. Spring Boot lets me focus on solving real problems (GeeksforGeeks).
- Monitoring and Management: The Actuator module is a gem. It gives you built-in tools to check your app’s health and performance with ready-to-use endpoints for monitoring and managing production environments (GeeksforGeeks).
Here’s a quick rundown of Spring Boot’s perks:
Benefit | What’s Cool About It |
---|---|
Simplicity | Auto-configuration and defaults make life easier. |
Microservices | Perfect for self-contained, scalable services. |
Built-In Server | No external server setup needed. |
No Boilerplate | Focus on real coding, less setup hassle. |
Monitoring | Actuator module for easy app health checks. |
For more details, dive into the spring boot documentation.
Getting to grips with Spring Boot has made my journey as a Java developer way smoother. It’s a powerful tool that can seriously boost your development game, especially if you’re just starting out.
Why Spring Boot Rocks for Java Developers
Spring Boot packs a punch with features that make life easier for Java developers. Let’s break down three of its coolest tricks: running apps on their own, keeping an eye on things with the Actuator module, and ditching the need for boilerplate code.
Apps That Run Solo
One of the best things about Spring Boot is its ability to create apps that can run all by themselves. No need for an external server to get things going. The server is baked right into the app, making deployment a breeze. This is a game-changer for microservices since each service can be deployed and scaled on its own.
Feature | Benefit |
---|---|
Runs Solo | Easy deployment |
Built-in Server | Perfect for microservices |
Want to see how Spring Boot stacks up against traditional Spring MVC? Check out our article on spring boot vs spring mvc.
Actuator Module: Your App’s Watchdog
Spring Boot comes with the Actuator module, which is like having a built-in watchdog for your apps. Actuator gives you ready-made endpoints to check how your app is doing. You can track metrics, monitor traffic, and tweak settings right in production.
Endpoint | What It Does |
---|---|
/actuator/health | Checks if your app is healthy |
/actuator/metrics | Shows stats like memory and CPU usage |
/actuator/env | Lists environment settings |
If you’re looking to lock down these endpoints, our guide on spring boot security has got you covered.
Bye-Bye Boilerplate Code
Spring Boot cuts down on the boring, repetitive code you usually need to write. Forget about generating code or messing with XML configurations. This lets you zero in on the important stuff—your business logic. Your codebase stays clean and easy to manage.
Feature | Benefit |
---|---|
No Boilerplate Code | Cleaner codebase |
No XML Configs | Simple setup |
For more nitty-gritty details, check out the official spring boot documentation.
By using these features, you can speed up your development and build solid, scalable apps. Curious about how Spring Boot can help with microservices? Dive into our detailed guide on spring boot microservices.
Getting Started with Spring Boot
Jumping into Spring Boot development? Knowing your tools and tricks can seriously boost your productivity. Let’s break down some essentials: Starter POMs, Microservices, and Testing.
Starter POMs: Your Best Friend
Spring Boot’s ‘opinionated’ starter POMs make your life easier by simplifying build configurations. These starters handle everything from embedding servers to setting up Spring’s JPA, giving you a head start. Take the spring-boot-starter-web
for example—perfect for web apps.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
You can add other dependencies as needed, balancing flexibility with simplicity. For more details, check out the Spring Boot documentation.
Microservices Made Easy
Spring Boot works great with Spring Cloud, making it a top pick for microservices. Each microservice can run on its own with its own database, making scaling and deployment a breeze.
Key features include:
- Centralized Config: Manage all your configurations in one place.
- API Gateway: Secure and streamline your requests.
- Scalability: Scale each microservice as needed.
For more on microservices, see our article on Spring Boot microservices.
Testing: Keep It Simple
Spring Boot offers a range of testing annotations to make testing easier. Some key ones are:
@SpringBootTest
: Loads the full application context.@WebMvcTest
: Focuses on the web layer.@DataJpaTest
: Specializes in JPA components.
You can also set different properties for different profiles to keep your tests from messing with your main app.
# application.properties
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
# application-test.properties
spring.datasource.url=jdbc:h2:mem:testdb
Using these annotations and profiles helps keep your code robust and maintainable. For more testing tips, visit our guide on Spring Boot security.
Master these basics, and you’ll be using Spring Boot’s powerful features to streamline your development and deliver top-notch applications.
Advanced Tricks with Spring Boot
Ready to level up your Spring Boot game? These tips will help you squeeze every bit of goodness out of your Spring Boot apps and make your coding life a breeze.
Custom App Settings
Spring Boot makes juggling different settings for different environments a cinch. You can whip up separate properties files for each environment, like application.properties
for production and application-test.properties
for testing. This way, you can tweak settings without messing up your main app.
Check out these sample configs:
# application.properties
server.port=8080
spring.datasource.url=jdbc:mysql://localhost/prod_db
# application-test.properties
server.port=8081
spring.datasource.url=jdbc:h2:mem:testdb
Switching between these settings is a snap. Just use the --spring.profiles.active
argument:
java -jar myapp.jar --spring.profiles.active=test
For more on configuring Spring Boot, take a peek at the spring boot documentation.
HTTP Requests with RestTemplate
Need to make HTTP requests? RestTemplate
has got your back. It’s your go-to for chatting with RESTful web services or APIs.
Here’s a quick example of a GET request:
import org.springframework.web.client.RestTemplate;
public class RestClient {
public static void main(String[] args) {
RestTemplate restTemplate = new RestTemplate();
String url = "https://api.example.com/data";
String response = restTemplate.getForObject(url, String.class);
System.out.println(response);
}
}
And for POST requests, try this:
String url = "https://api.example.com/data";
Data data = new Data("example");
Data response = restTemplate.postForObject(url, data, Data.class);
System.out.println(response);
RestTemplate
makes HTTP requests a walk in the park, so you can focus on the fun stuff.
Code Coverage and Testing Tools
Keeping your code well-tested is a must for a solid app. Tools like SonarQube and Jacoco help you see how much of your code is covered by tests and give you insights into your testing.
Here’s a quick rundown of some popular tools:
Tool | Features | Integration |
---|---|---|
Jacoco | Measures code coverage, generates reports | Maven, Gradle |
SonarQube | Comprehensive code quality analysis | Jenkins, Bamboo |
Cobertura | Code coverage reporting | Ant, Maven |
Aim for high code coverage to make sure your code is solid. For example, here’s how you can integrate Jacoco into a Maven project:
<build>
<plugins>
<plugin>
<groupId>org.jacoco</groupId>
<artifactId>jacoco-maven-plugin</artifactId>
<version>0.8.7</version>
<executions>
<execution>
<goals>
<goal>prepare-agent</goal>
</goals>
</execution>
<execution>
<id>report</id>
<phase>test</phase>
<goals>
<goal>report</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
For more on testing Spring Boot apps, check out this Medium article.
By using these tricks, you can make your Spring Boot apps more flexible, reliable, and easier to maintain. For more tips and detailed guides, explore our articles on spring boot security and spring boot microservices.