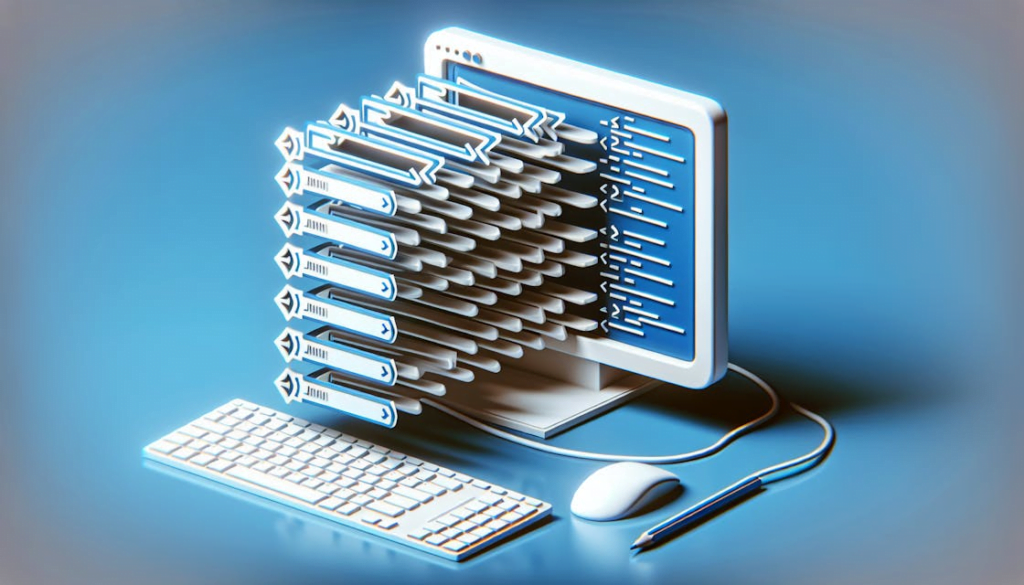
Getting the Hang of Java Arrays
Introduction to Arrays
When I first dipped my toes into Java, arrays were a game-changer. Think of arrays as magic boxes that let you store a bunch of values in one go, instead of juggling a ton of separate variables. They’re like the Swiss Army knife of Java, holding a fixed number of values of a specific type, like integers or strings.
Arrays make life easier, especially when dealing with lots of data. Imagine needing to handle 100 different numbers. Without arrays, you’d be stuck declaring 100 separate variables. With arrays, you just stash all those numbers in one neat package.
Declaration and Initialization
Getting arrays up and running in Java was one of the first things I tackled. Here’s a quick rundown:
Declaring an Array
To declare an array, you slap some square brackets onto your variable type. You can stick the brackets after the data type or the variable name. Both ways work:
int[] myArray;
int myArray[];
Both lines do the same thing—they set up a reference to an integer array.
Initializing an Array
Declaring an array is just the first step. To actually create the array, you need to specify its type and how many elements it can hold. For example, to make an integer array with room for 10 integers, you’d write:
myArray = new int[10];
You can also do it all in one go:
int[] myArray = new int[10];
Filling Up the Array
Once your array is set up, you can start filling it with values. Each spot in the array is accessed using an index, starting at 0. Here’s how you’d populate the array:
myArray[0] = 1;
myArray[1] = 2;
myArray[2] = 3;
// and so on...
Here’s a quick look at what the array would look like:
Index | Value |
---|---|
0 | 1 |
1 | 2 |
2 | 3 |
… | … |
9 | 10 |
Key Points to Remember
- Fixed Size: Once you create an array, its size is set in stone. Try to add more elements than it can hold, and you’ll get an
ArrayIndexOutOfBoundsException
. - Contiguous Memory: Arrays store values in a continuous block of memory, making it quick to access elements.
- Type-Specific: Every element in an array must be of the same type.
If you need something more flexible, check out ArrayList
, which we’ll cover in the Arrays vs. ArrayList in Java section.
Grasping arrays is a must for efficient Java programming. With this under your belt, you can move on to more advanced stuff like Java loops and Java methods. Happy coding!
Working with Java Arrays
Hey there, budding Java whiz! Let’s chat about how to mess around with Java arrays. If you’re just starting out, getting the hang of these basics will make your coding life a whole lot easier.
Accessing Array Elements
So, Java arrays are like a row of lockers, each with its own number. The first locker is number 0, the second is 1, and so on. Here’s a quick peek:
int[] myArray = {10, 20, 30, 40, 50};
System.out.println(myArray[0]); // Outputs 10
System.out.println(myArray[2]); // Outputs 30
Want to check out all the lockers? Use a for
loop. It’s like having a master key to peek inside each one:
for (int i = 0; i < myArray.length; i++) {
System.out.println("Element at index " + i + ": " + myArray[i]);
}
Here’s what you’d see:
Index | Element |
---|---|
0 | 10 |
1 | 20 |
2 | 30 |
3 | 40 |
4 | 50 |
Modifying Array Elements
Changing the stuff inside your lockers? Easy peasy. Just point to the locker number and swap out the contents:
myArray[1] = 25; // Changes the second element (index 1) to 25
System.out.println(myArray[1]); // Outputs 25
Need to change a bunch of lockers at once? Use a loop to double everything inside:
for (int i = 0; i < myArray.length; i++) {
myArray[i] = myArray[i] * 2;
}
After that, your array looks like this:
Index | Element |
---|---|
0 | 20 |
1 | 50 |
2 | 60 |
3 | 80 |
4 | 100 |
If you’re itching to dive deeper into arrays, like using them in methods or classes, check out our java methods and java inheritance sections.
Playing around with arrays is something you’ll do a lot in Java. For more cool tips and tricks, swing by our java tutorial section. Happy coding!
Sorting and Searching in Arrays
Sorting and searching in arrays are must-know skills for any Java developer. Let’s break down how to handle these tasks in Java.
Sorting Arrays in Java
Sorting arrays in Java is a breeze with the Arrays.sort()
method. This handy method can sort arrays of different data types like int
, long
, char
, and float
. It’s part of the java.util.Arrays
class and has a time complexity of O(N log N) and an auxiliary space complexity of O(1) (GeeksforGeeks).
Here’s how you can sort an array of integers in ascending order:
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[] numbers = {5, 2, 9, 1, 5, 6};
Arrays.sort(numbers);
System.out.println(Arrays.toString(numbers)); // Output: [1, 2, 5, 5, 6, 9]
}
}
Want to sort an array in descending order? Use the reverseOrder()
method from the Collections
class. It first sorts the array in ascending order and then flips it:
import java.util.Arrays;
import java.util.Collections;
public class Main {
public static void main(String[] args) {
Integer[] numbers = {5, 2, 9, 1, 5, 6};
Arrays.sort(numbers, Collections.reverseOrder());
System.out.println(Arrays.toString(numbers)); // Output: [9, 6, 5, 5, 2, 1]
}
}
Searching for Elements
Finding elements in an array is a snap with the Arrays.binarySearch()
method. Just make sure your array is sorted in ascending order first. If the element is found, it returns the index; if not, you get a negative value.
Check this out:
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[] numbers = {1, 2, 5, 5, 6, 9};
int index = Arrays.binarySearch(numbers, 5);
System.out.println(index); // Output: 2
}
}
You can use the same method for searching strings:
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
String[] words = {"apple", "banana", "cherry", "date"};
int index = Arrays.binarySearch(words, "cherry");
System.out.println(index); // Output: 2
}
}
For more Java goodness, dive into topics like java loops, java methods, and java inheritance to expand your coding toolkit.
Arrays vs. ArrayList in Java
When you’re dealing with collections of data in Java, you’ve got two main players: Arrays and ArrayLists. Each has its own quirks and perks. Let’s break it down and see what makes them tick.
Array Basics
Arrays are the old-school way to store a bunch of elements of the same type. Here’s what you need to know:
- Fixed Size: Once you set an array’s size, that’s it. No take-backs. You gotta know how many elements you need upfront. For example,
int[] myArray = new int[10];
sets up an array for 10 integers. - Static: Arrays don’t change size. They’re like that friend who never changes their order at a restaurant.
- Versatile Storage: Arrays can hold both primitive types (like
int
,char
, etc.) and objects. Handy, right? - Speed: Arrays are usually quicker than ArrayLists for fixed-size data because they don’t have the overhead of resizing.
Here’s a quick example of setting up an array:
int[] numbers = {1, 2, 3, 4, 5};
ArrayList Perks
ArrayLists, part of the java.util
package, are like arrays but with more flexibility and cool features:
- Dynamic Size: ArrayLists can grow and shrink as needed. No need to know the size ahead of time. For example,
ArrayList<Integer> myList = new ArrayList<>();
sets up an ArrayList that can change size on the fly. - Extra Features: ArrayLists come with a bunch of handy methods like
indexOf()
,remove()
, and more. Arrays don’t have these tricks up their sleeves. - Object-Only Storage: ArrayLists can only store objects. If you add a primitive type, Java automatically converts it to its wrapper class (e.g.,
int
becomesInteger
). - Under the Hood: ArrayLists are backed by arrays. When they fill up, they create a new, bigger array and copy everything over. This resizing can slow things down a bit.
Here’s how you can set up an ArrayList:
ArrayList<Integer> numbersList = new ArrayList<>();
numbersList.add(1);
numbersList.add(2);
numbersList.add(3);
Quick Comparison
Feature | Array | ArrayList |
---|---|---|
Size | Fixed | Dynamic |
Can store | Primitives and Objects | Only Objects |
Extra Features | No | Yes (e.g., indexOf() , remove() ) |
Speed | Faster for fixed size | Slower due to resizing |
For more tips on using arrays and ArrayLists in Java, check out our Java tutorial and explore various Java methods to manipulate these data structures effectively.