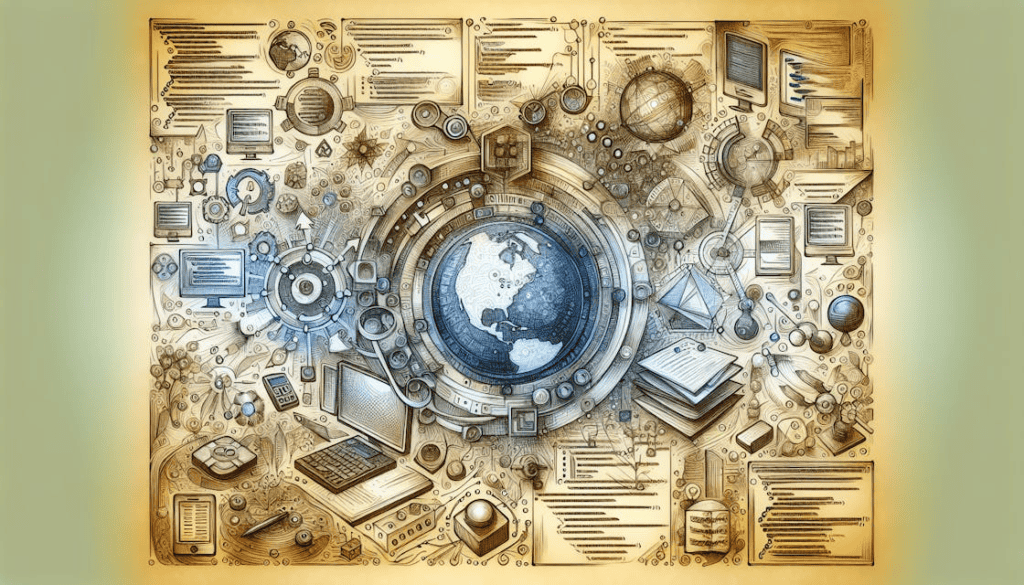
Getting the Hang of Java Methods
What Makes Up a Java Method?
When I first dipped my toes into Java programming, figuring out the nuts and bolts of a method was a game-changer. A method in Java is basically a chunk of code that does something specific. Unlike languages like C, C++, and Python, every method in Java has to be part of a class (GeeksforGeeks). Let’s break down what makes up a Java method:
- Modifier: Tells you who can use the method, like
public
,private
, orprotected
. - Return Type: The type of data the method gives back. If it doesn’t give back anything, it uses
void
. - Method Name: The name you use to call the method. It should tell you what the method does.
- Parameter List: The data you pass into the method, wrapped in parentheses.
- Exception List: Any exceptions the method might throw.
- Method Body: The actual code that does the work.
Example:
public int addNumbers(int a, int b) {
return a + b;
}
In this example:
public
is the modifier.int
is the return type.addNumbers
is the method name.(int a, int b)
is the parameter list.return a + b;
is the method body.
For more details on method structure, check out our java tutorial.
Why Methods Matter
Methods are a big deal in Java programming for a bunch of reasons:
- Code Reusability: Write a method once, use it anywhere in your code. It’s like having a magic wand.
- Modularity: Break down big problems into smaller, bite-sized tasks. Each method does one thing, making your code easier to read and manage.
- Ease of Testing: You can test methods one by one, making debugging a breeze.
- Abstraction: Methods let you hide the messy details and show only the useful stuff, making your code cleaner and easier to understand.
For example:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public int subtract(int a, int b) {
return a - b;
}
public int multiply(int a, int b) {
return a * b;
}
}
In this Calculator
class, I’ve got methods for add
, subtract
, and multiply
. Each one does its own thing, making the code modular and reusable.
Getting a handle on methods is just the start. As you get better, you can dive into more advanced stuff like java inheritance and java loops to level up your programming game.
Types of Java Methods
Hey there, budding Java developer! Let’s break down the different types of methods in Java. There are two main categories: predefined methods and user-defined methods.
Predefined Methods
Predefined methods, also known as standard library methods or built-in methods, are already defined within Java class libraries. These methods are like your go-to tools in a toolbox—always ready to use.
Here are some common examples:
Method | What It Does |
---|---|
length() | Gets the length of an array or a string. |
equals() | Compares two strings to see if they’re the same. |
compareTo() | Compares two strings alphabetically. |
sqrt() | Returns the square root of a number. |
These methods save you from reinventing the wheel. For instance, instead of writing your own code to find the length of a string, you can just use length()
. Check out more about using loops with these methods in our java loops tutorial.
User-defined Methods
User-defined methods are the ones you create to do specific tasks that aren’t covered by predefined methods. Think of them as custom tools you make for your own toolbox.
Here’s a simple example:
public class MyClass {
// Custom method
public void displayMessage() {
System.out.println("Hello, Java world!");
}
public static void main(String[] args) {
MyClass obj = new MyClass();
// Calling the custom method
obj.displayMessage();
}
}
In this example, displayMessage()
is a custom method that prints a message to the console. You call it by creating an instance of the class and then invoking the method.
Custom methods let you tailor your code to fit your needs, making it more modular and easier to maintain. For more advanced stuff, check out our guide on java inheritance.
Understanding these types of methods and how to use them will help you write better, more efficient Java programs. For more learning, visit our comprehensive java tutorial.
Cracking Java Methods
Getting the hang of Java methods is like learning the secret sauce for writing smooth, efficient code. Let’s break it down into bite-sized pieces, focusing on the modifier, return type, method name, and parameter list.
Modifier and Return Type
First up, we have the modifier and return type. The modifier tells us who can use the method. Think of it like a bouncer at a club. If it’s public
, everyone gets in. If it’s private
, only the VIPs (inside the class) are allowed. (CodeGym)
The return type is what the method gives back. If it’s void
, it’s like a chatty friend who doesn’t expect a reply. Here’s a quick cheat sheet:
Return Type | What It Gives Back |
---|---|
int | An integer (like 42) |
double | A double (like 3.14) |
boolean | True or false |
void | Nada, zilch, nothing |
Pick the return type that matches what your method is supposed to hand over.
Method Name and Parameter List
Next, we have the method name and parameter list. The method name is how you call it over. Java likes method names to be verbs in lowercase, or a combo of words starting with a verb in lowercase followed by other words with their first letters capitalized. (Oracle Java Methods)
For example:
public int calculateSum(int a, int b) {
return a + b;
}
Here, calculateSum
is the method name.
The parameter list is like the guest list for a party. These are the variables that get passed into the method. The method signature is the method name plus the parameter list, minus the return type and exceptions. (GeeksforGeeks)
Here’s a method with a couple of parameters:
public void printDetails(String name, int age) {
System.out.println("Name: " + name + ", Age: " + age);
}
In this example, printDetails
is the method name, and it takes two guests: a String
named name
and an int
named age
.
By nailing down these components, you’ll be crafting well-structured methods in Java in no time. For more Java goodness, check out our java tutorial and other cool reads like java loops and java arrays.
Java Method Best Practices
Reusability of Methods
In Java, making methods reusable is a game-changer. Reusability means you can use a method multiple times without rewriting it, saving you time and keeping your code neat and tidy.
Java methods can perform tasks without returning anything, making it easy to reuse code without retyping. For example, if you have a method that adds two numbers, you can call this method whenever you need it, instead of writing the same code over and over.
public class Calculator {
public int sum(int a, int b) {
return a + b;
}
}
Here, the sum
method can be called with different arguments, promoting reusability.
Java also supports method overloading, allowing methods in a class to share the same name as long as they have different parameter lists. Overloaded methods are distinguished by the number and type of arguments passed in.
public class Calculator {
public int sum(int a, int b) {
return a + b;
}
public double sum(double a, double b) {
return a + b;
}
}
In this example, the sum
method is overloaded to handle both integers and doubles.
Naming Conventions for Methods
Good naming conventions are key to clear and readable code. In Java, method names should be descriptive and follow camelCase notation, where the first word is lowercase and each subsequent word starts with an uppercase letter.
Here are some tips for naming methods:
- Descriptive Names: Method names should clearly describe what the method does. For example, a method that adds two numbers could be named
calculateSum
. - Verb-Noun Phrases: Method names often start with a verb followed by a noun. Examples include
printMessage
,calculateSum
, orfetchData
. - Consistency: Consistent naming conventions across your codebase help in understanding and maintaining the code. Stick to the same pattern for naming methods throughout your project.
public class UserProfile {
public void printUserInfo() {
// Code to print user information
}
public int calculateUserAge(int birthYear) {
// Code to calculate user age
return 2023 - birthYear;
}
}
In the example above, the method names printUserInfo
and calculateUserAge
follow the verb-noun structure and camelCase notation, making the code easy to read and understand.
For more tips and best practices on Java programming, check out our java tutorial and learn about java loops and java arrays. You can also explore advanced topics like java inheritance.